Why Dead Code Is Hurting Your Team — and How to Fix It
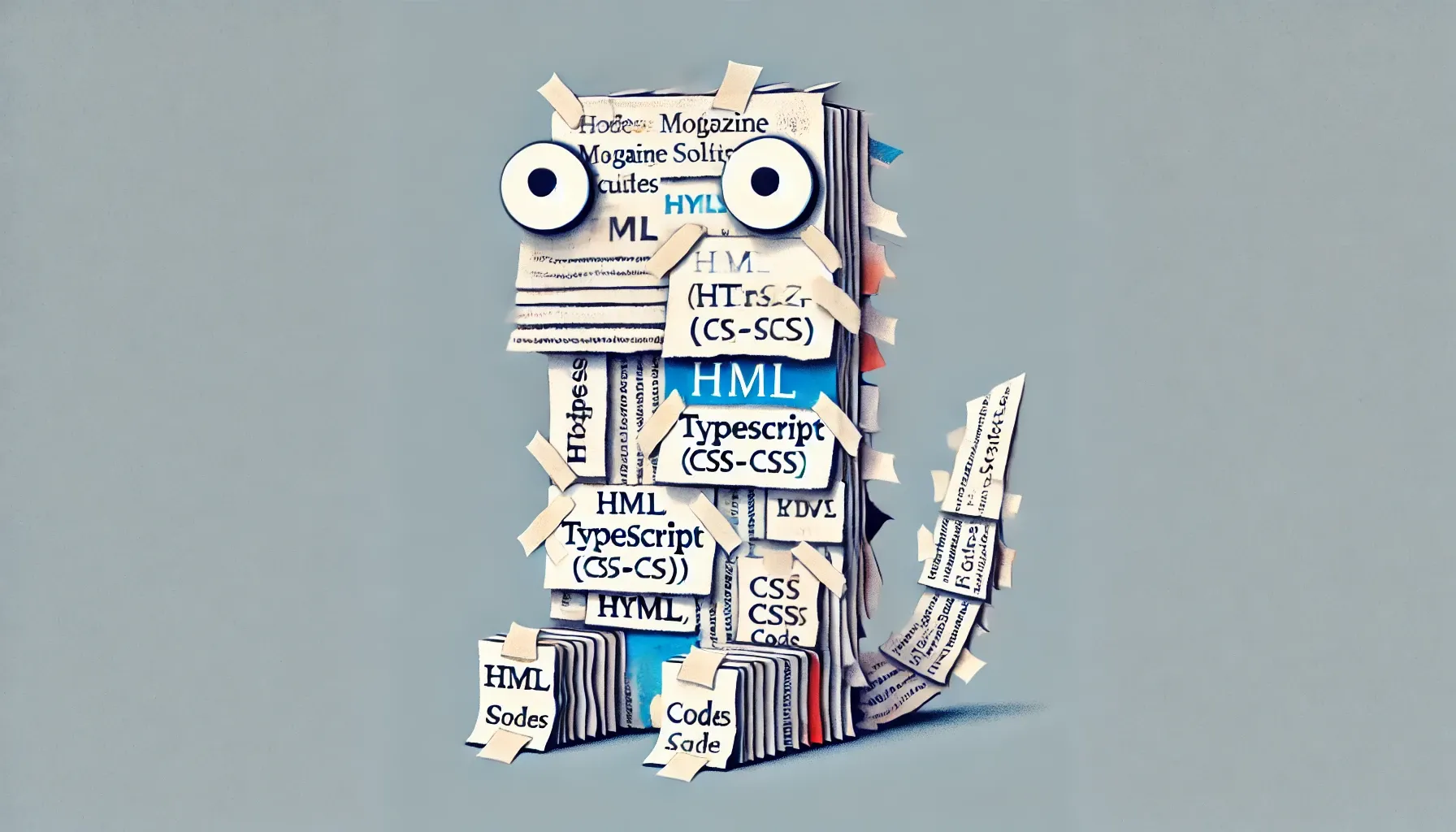
Dead code is a silent form of technical debt, and it’s slowing your team down. Dead code increases the complexity of your codebase which makes it harder to implement new features, improve existing functionality, or refactor code efficiently. Ignoring it can lead to slower development cycles, frustrated developers, and unnecessary costs.
What Is Dead Code?
Dead code is any code in your codebase that isn’t actively used in your application. This could range anywhere from unused files all the way down to unused exports, dependencies, or even enum properties. While it might seem harmless at first, dead code contributes to clutter, confusion, and wasted effort. Removing it is crucial to maintaining a clean, efficient, and scalable codebase.
The Hidden Costs of Dead Code
The impact of dead code is often subtle but highly consequential. To understand its effects, let’s look at a simple example in TypeScript:
type Params = {
value: number;
};
function unusedFunction(params: Params) {
// does things
}
function realFunction(params: Params) {
// does things
}
Now, imagine you need to change the value property from a number
to a boolean
. This small update forces you to modify both realFunction
and unusedFunction
—even though unusedFunction
isn’t being used anywhere in your code.
If unusedFunction
is complex, you’ll spend critical dev cycles deciphering its purpose, dependencies, and downstream implications. Worse, you might need input from other developers to understand its original intent. All this extra work delays your task, increases development costs, and risks missing tight deadlines.
The Bigger Picture: Dead Code Across Your Codebase
Removing dead code requires a deliberate and systematic approach. Here are two main strategies to consider:
1. Manual Auditing
One way to remove dead code is by manually reviewing your codebase. This approach works for smaller projects but quickly becomes impractical for larger codebases. It’s time-consuming, error-prone, and may lead to accidentally removing active code or missing significant dead code paths.
2. Automated Tools
For larger projects, automating the detection and removal of dead code is a game-changer. Tools like remove-unused simplify this process by analyzing your TypeScript codebase, identifying unused files, and providing actionable reports.
remove-unused
works by inspecting your Typescript codebase. It builds your project’s dependency graph by looking at all of your code’s import and require statements. It then flags files that aren’t referenced anywhere. This accounts for aliases or custom paths, and ensures unused code is accurately flagged.
remove-unused
is also designed to work seamlessly with modern frameworks like Next.js. It automatically recognizes critical directories such as app or pages and even supports MDX files.
Using tools like remove-unused
to automatically remove dead code will save your team time, reduce errors, and allow your to focus on solving core business problems instead of wrestling with outdated code.
Why Dead Code Shouldn’t Be Ignored
Dead code isn’t just an annoyance—it’s a productivity killer. It adds complexity to your codebase, increases maintenance costs, and slows down feature delivery. Addressing dead code proactively improves code quality, boosts team morale, and accelerates development.
Start cleaning up your codebase today. Whether you use manual auditing for small projects or automated tools like remove-unused for larger ones, taking action now will set your team up for success.
Ready to eliminate dead code? Learn more at removeunused.com.